Rails Testing: The Battle of Factories vs Fixtures when using RSpec
A comparison of the pros/cons of using factories vs fixtures for testing Ruby on Rails applications with RSpec.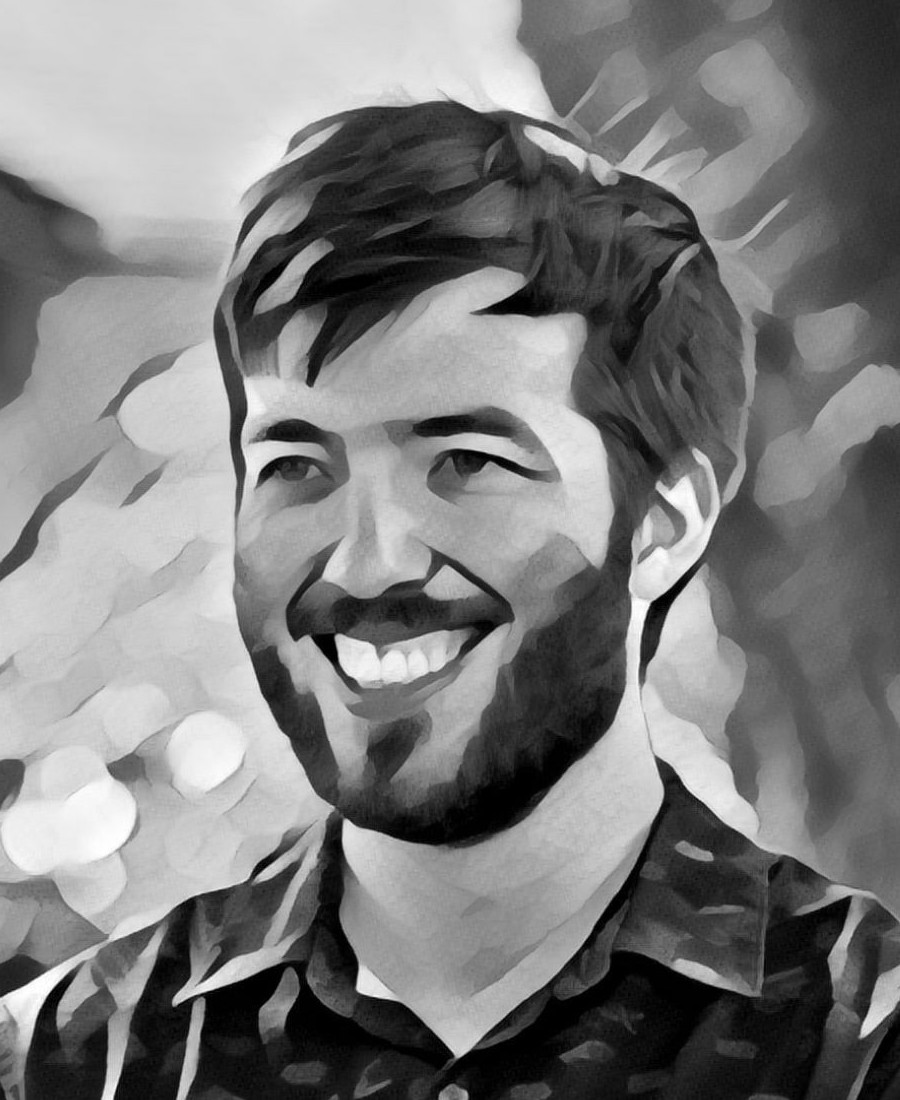
Alex McDermid
August 18th, 2023RSpec is a behavior-driven development (BDD) testing framework for the Ruby programming language. BDD emphasizes writing tests in a more natural language style, making it easier to read and understand the test cases. RSpec provides a rich DSL (domain-specific language) to describe the behavior of Ruby code in a readable manner. Its syntax and structure allow developers to write tests that closely resemble regular English, making it easier for non-technical stakeholders to understand the specifications and behaviors of the system.
In Rails, RSpec can replace the default Minitest framework, giving developers a more extensive toolset for writing and organizing tests. It integrates well with other testing tools and libraries.
Fixtures
Fixtures are a way of populating your testing database with predefined data before tests run. They're provided by Rails by default. Fixtures are defined in YAML files, and Rails will load these files to set up the test database with a known state.
For instance, if you have a model User, you might have a fixture file named users.yml:
While fixtures provide a quick way to have a consistent test database state, they can be cumbersome when dealing with complex or related data, or when needing to create unique or randomized data for every test.
This is because Fixtures provide static test data which can become cumbersome when managing complex model relationships or requiring dynamic, unique, or randomized data for tests. This static nature can lead to challenges in maintenance, especially with evolving application structures and relationships. Tools like FactoryBot offer more flexibility in such scenarios, enabling dynamic data generation and easier handling of model associations.
Pros
- Speed: Fixtures can be faster because they load data directly into the database at the start of a test suite.
- Simplicity: Written in YAML or CSV, they are simple and straightforward.
- Global Availability: Once loaded, they are available for all tests.
- Rails Native: Built into Rails, no additional gem installation or maintenance required.
Cons
- Rigidity: Less flexibility to customize data on-the-fly for specific test cases.
- Flat Files: All data is stored in flat files, which can be a challenge for managing complex or extensive data setups.
- Global State: Since fixtures create a global state for tests, tests can become interdependent if not written carefully.
- Transition Effort: Shifting to fixtures from another system, like FactoryBot, requires significant effort. This includes writing fixture files for every model and modifying existing tests to adopt the fixture data.
- Representation of Relationships: Complex relationships and polymorphic associations can be challenging to represent in YAML/CSV format used by fixtures.
- State Pollution: Fixtures introduce a global state for tests, which might lead to unintended interactions between tests. This can affect the consistency of test environments, leading to unpredictable test outcomes due to data dependencies across tests.
Factories
FactoryBot is a library (Gem) for setting up Ruby objects as test data. Unlike fixtures, which provide static predefined data, FactoryBot offers a more dynamic and flexible approach. It uses the concept of factories to define the blueprint of objects and allows you to easily override, extend, or randomize the generated data.
Factories defined with FactoryBot are usually more adaptable and concise than fixtures. They can be easily combined, extended, and customized to fit different scenarios. This is particularly useful in tests where data needs to respect certain validations, or when you want to simulate various scenarios without having to redefine the entire data set.
In the context of RSpec, FactoryBot often feels more natural as it follows the same BDD principles. Instead of setting up a static state for your tests like fixtures do, FactoryBot generates the data you need on-demand, making it clear within the test what kind of data is being tested.
Here's an example of a factory for the User model:
With this factory, in an RSpec test, you can create a user like so:
Pros
- Flexibility: Easily customize and override attributes for specific test cases.
- Laziness: FactoryBot lets you build objects without persisting them to the database until necessary.
- Associations: Simple declaration for associations and building associated objects.
- Traits: Use traits to quickly generate variations of a base object.
- Sequences: Handy for attributes that need to be unique, such as emails or usernames.
Cons
- Speed: Depending on your usage, FactoryBot can be slower than fixtures because it can involve more database writes and object instantiations.
- Complexity: Over time, factories can grow complex, especially with nested attributes and callbacks.
Incorporating Gems
Thinking about incorporating third-party gems like FactoryBot into your existing Rails application? Check out our blog post Gem Detective: A How-to (and How-to-not) on Incorporating Gems at Harled , written by our Development Manager, Vlad Hociota. The article provides a deep dive into the process of identifying, evaluating, and implementing new Ruby gems, sharing our real-world experiences, learnings, and challenges.
Wrap Up
While RSpec provides the structure and domain-specific language for defining and running tests, both fixtures and FactoryBot factories offer ways to manage test data. Fixtures provide static, predefined data that is fast while FactoryBot offers a more dynamic and customizable approach, making it easier to set up varied scenarios within tests.
For those starting with a simple data structure, using fixtures can be a straightforward way to manage test data. They can be a good entry point for teams, especially when dealing with a well-defined and stable data model. However, as the application grows in complexity, and the need for more intricate testing scenarios arises, the rigidity of fixtures might become a limitation.
Transitioning from fixtures to factories (such as FactoryBot) can be a natural progression, especially when needing to simulate various complex scenarios without having to redefine the entire data set. Factories provide a way to efficiently handle dynamic, unique, or randomized data for tests, as well as manage complex model associations, giving them an edge in more sophisticated testing environments.
The choice between Fixtures and FactoryBot isn't absolute. Depending on the complexity of the application, the dynamics of the test data needed, and the familiarity of the development team with the tools, one might be chosen over the other. It's essential for developers to understand the strengths and weaknesses of both and pick what aligns best with their testing philosophy and application needs.
Interested in joining a mission-driven team with a passion for Ruby on Rails? If so, please take a moment to look at our open positions!.
About the author
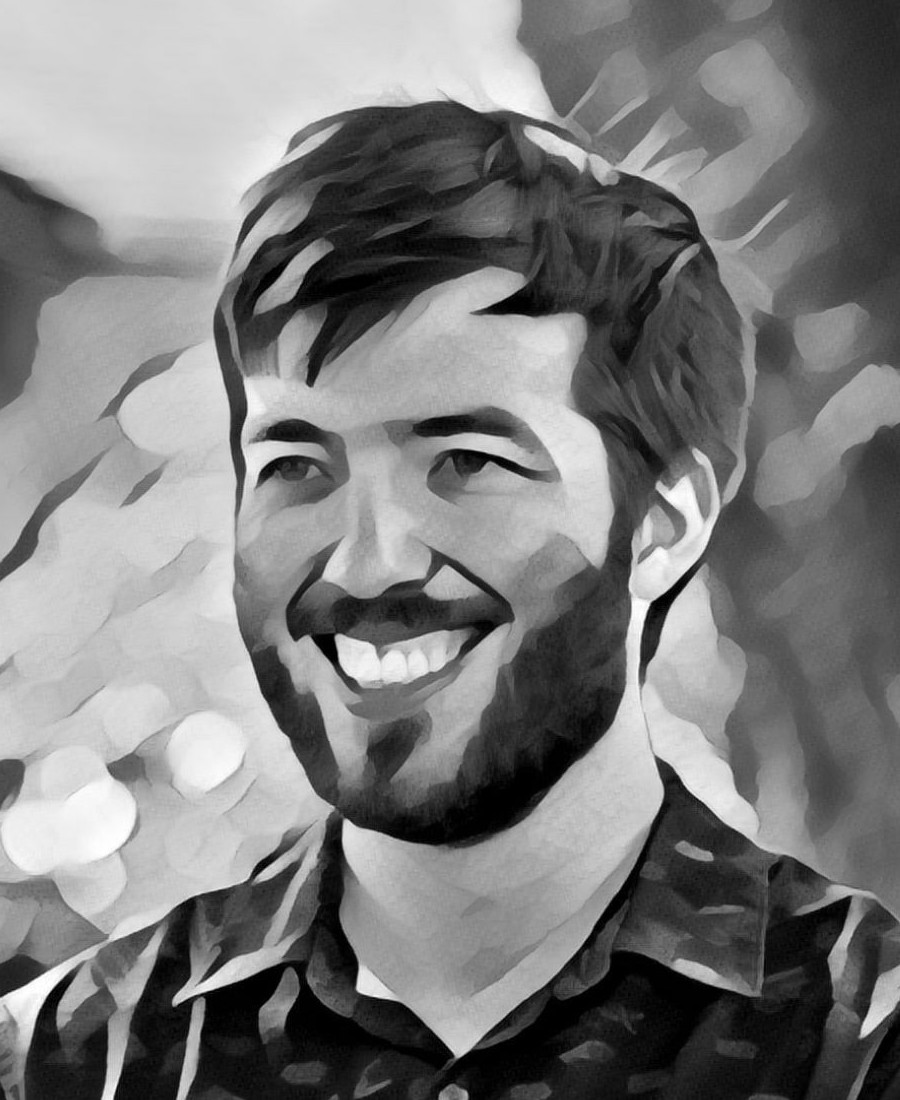
Alex is a Full Stack Developer based out of Surrey, BC.